Basic query for C and C++ code¶
Learn to write and run a simple CodeQL query using Visual Studio Code with the CodeQL extension.
For information about installing the CodeQL extension for Visual Studio code, see “Installing CodeQL for Visual Studio Code.”
About the query¶
The query we’re going to run performs a basic search of the code for if
statements that are redundant, in the sense that they have an empty then branch. For example, code such as:
if (error) { }
Finding a CodeQL database to experiment with¶
Before you start writing queries for C/C++ code, you need a CodeQL database to run them against. The simplest way to do this is to download a database for a repository that uses C/C++ directly from GitHub.com.
- In Visual Studio Code, click the QL icon
in the left sidebar to display the CodeQL extension.
- Click From GitHub or the GitHub logo
at the top of the CodeQL extension to open an entry field.
- Copy the URL for the repository into the field and press the keyboard Enter key. For example, https://github.com/protocolbuffers/protobuf.
- Optionally, if the repository has more than one CodeQL database available, select
cpp
to download the database created from the C/C++ code.
Information about the download progress for the database is shown in the bottom right corner of Visual Studio Code. When the download is complete, the database is shown with a check mark in the Databases section of the CodeQL extension (see screenshot below).
Running a quick query¶
The CodeQL extension for Visual Studio Code adds several CodeQL: commands to the command palette including Quick Query, which you can use to run a query without any set up.
From the command palette in Visual Studio Code, select CodeQL: Quick Query.
After a moment, a new tab quick-query.ql is opened, ready for you to write a query for your currently selected CodeQL database (here a
cpp
database). If you are prompted to reload your workspace as a multi-folder workspace to allow Quick queries, accept or create a new workspace using the starter workflow.
In the quick query tab, delete
select ""
and paste the following query beneath the import statementimport cpp
.from IfStmt ifstmt, BlockStmt block where ifstmt.getThen() = block and block.getNumStmt() = 0 select ifstmt, "This 'if' statement is redundant."
Save the query in its default location (a temporary “Quick Queries” directory under the workspace for
GitHub.vscode-codeql/quick-queries
).Right-click in the query tab and select CodeQL: Run Query on Selected Database. (Alternatively, run the command from the Command Palette.)
The query will take a few moments to return results. When the query completes, the results are displayed in a CodeQL Query Results view, next to the main editor view.
The query results are listed in two columns, corresponding to the expressions in the
select
clause of the query. The first column corresponds to the expressionifstmt
and is linked to the location in the source code of the project whereifstmt
occurs. The second column is the alert message.
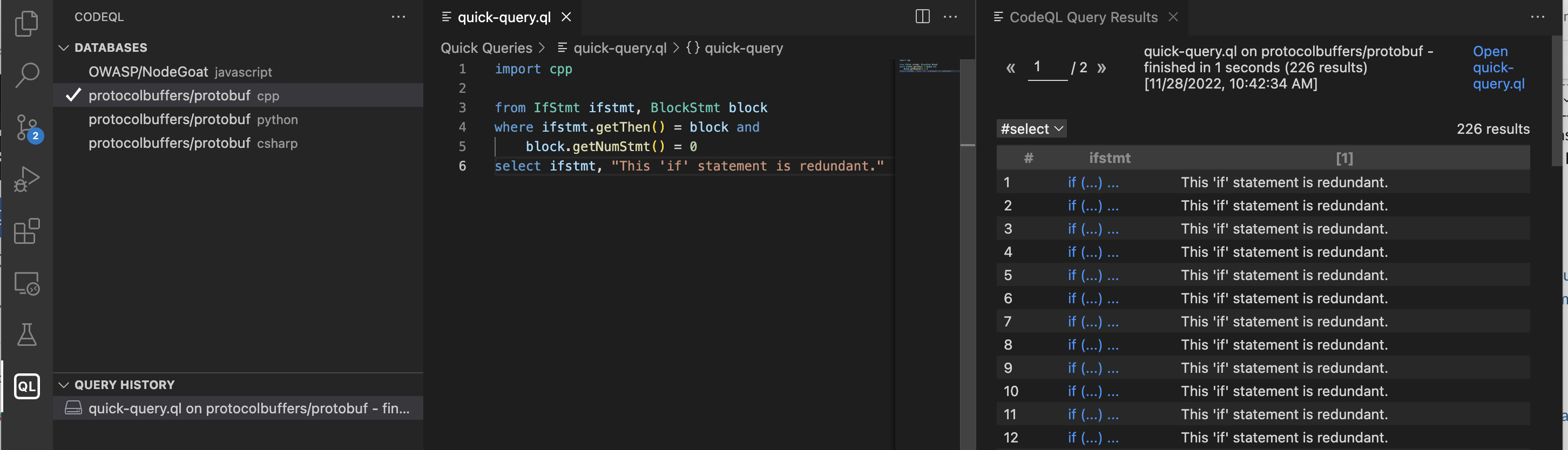
If any matching code is found, click a link in the ifstmt
column to open the file and highlight the matching if
statement.
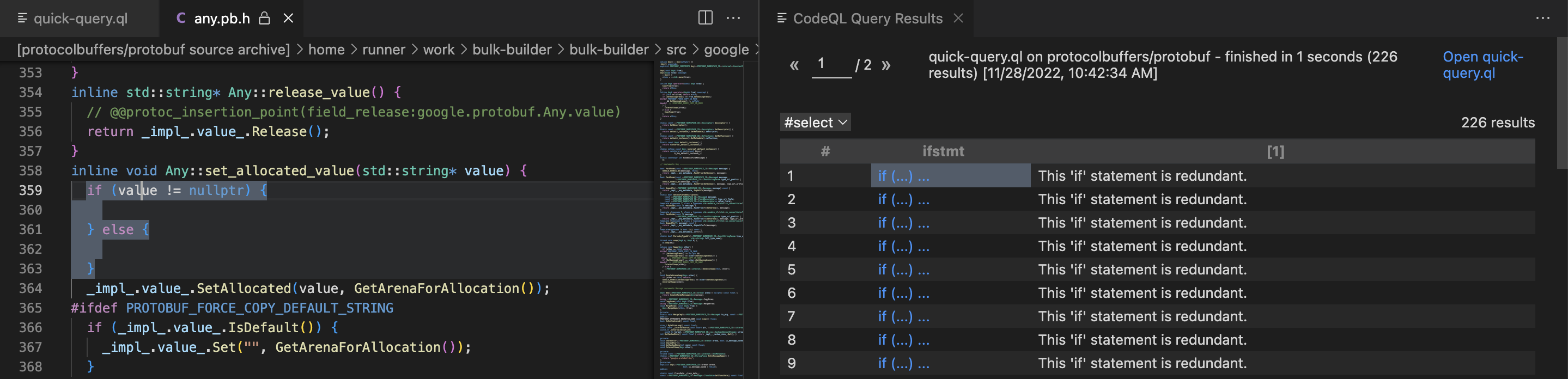
Note
If you want to move your experimental query somewhere more permanent, you need to move the whole
Quick Queries
directory. The directory is a CodeQL pack with aqlpack.yml
file that defines the content as queries for C/C++ CodeQL databases. For more information about CodeQL packs, see “Managing CodeQL query packs and library packs.”
About the query structure¶
After the initial import
statement, this simple query comprises three parts that serve similar purposes to the FROM, WHERE, and SELECT parts of an SQL query.
Query part | Purpose | Details |
---|---|---|
import cpp |
Imports the standard CodeQL libraries for C/C++. | Every query begins with one or more import statements. |
from IfStmt ifstmt, BlockStmt block |
Defines the variables for the query.
Declarations are of the form:
<type> <variable name> |
We use:
|
where ifstmt.getThen() = block and block.getNumStmt() = 0 |
Defines a condition on the variables. |
|
select ifstmt, "This 'if' statement is redundant." |
Defines what to report for each match.
|
Reports the resulting if statement with a string that explains the problem. |
Extend the query¶
Query writing is an inherently iterative process. You write a simple query and then, when you run it, you discover examples that you had not previously considered, or opportunities for improvement.
Remove false positive results¶
Browsing the results of our basic query shows that it could be improved. Among the results you are likely to find examples of if
statements with an else
branch, where an empty then
branch does serve a purpose. For example:
if (...) {
...
} else if (!strcmp(option, "-verbose")) {
// nothing to do - handled earlier
} else {
error("unrecognized option");
}
In this case, identifying the if
statement with the empty then
branch as redundant is a false positive. One solution to this is to modify the query to ignore empty then
branches if the if
statement has an else
branch.
To exclude if
statements that have an else
branch:
Edit your query and extend the
where
clause to include the following extra condition:and not ifstmt.hasElse()
The
where
clause is now:where ifstmt.getThen() = block and block.getNumStmt() = 0 and not ifstmt.hasElse()
Re-run the query.
There are now fewer results because
if
statements with anelse
branch are no longer reported.